How To Use Exit Function In Dev C++
- The C Standard Library
- C Standard Library Resources
C Language: exit function (Exit from Program) In the C Programming Language, the exit function calls all functions registered with atexit and terminates the program. File buffers are flushed, streams are closed, and temporary files are deleted. May 25, 2011 For 'exit', you need a '#include ' at the beginning of your program. There is no 'clrscr' function in any standard that I know of. I'm not sure what documentation you found that in, but 'clrscr' is neither a C nor a Windows console function. Use the atexit function to specify actions that execute prior to program termination. No global static objects initialized prior to the call to atexit are destroyed prior to execution of the exit-processing function. Return statement in main. Issuing a return statement from main is functionally equivalent to calling the exit function.
Upgrade to shiny stoves, fancy food prep stations, and more to ensure all customers get three-star service!PRIZE WHEELS!Introducing Weldon Brownie’s brand new game show – Spin to Win! Spin a free Prize Wheel every day to win prizes like Gold, Prep Recipes, VIP Tickets, Auto Chefs, Outfits, Pets, and more!OUTFITS & PETS!Dress up Flo in fun outfits themed for each Show, and equip Pets to help you out in the kitchen by auto-serving Prep Recipes!SERIES FINALES!Want to test your skill? Beat every episode in a Venue with 4 or more Stars to unlock!TRIAL OF STYLE!Try your luck in the Trial of Style! Face a gauntlet of challenging levels in a Venue’s Series Finale – experts only! Free download cooking games apk games.
Nov 10, 2016 This feature is not available right now. Please try again later. The C library function void exit(int status) terminates the calling process immediately. Any open file descriptors belonging to the process are closed and any children of the process are inherited by process 1, init, and the process parent is sent a SIGCHLD signal. This function does not return any.
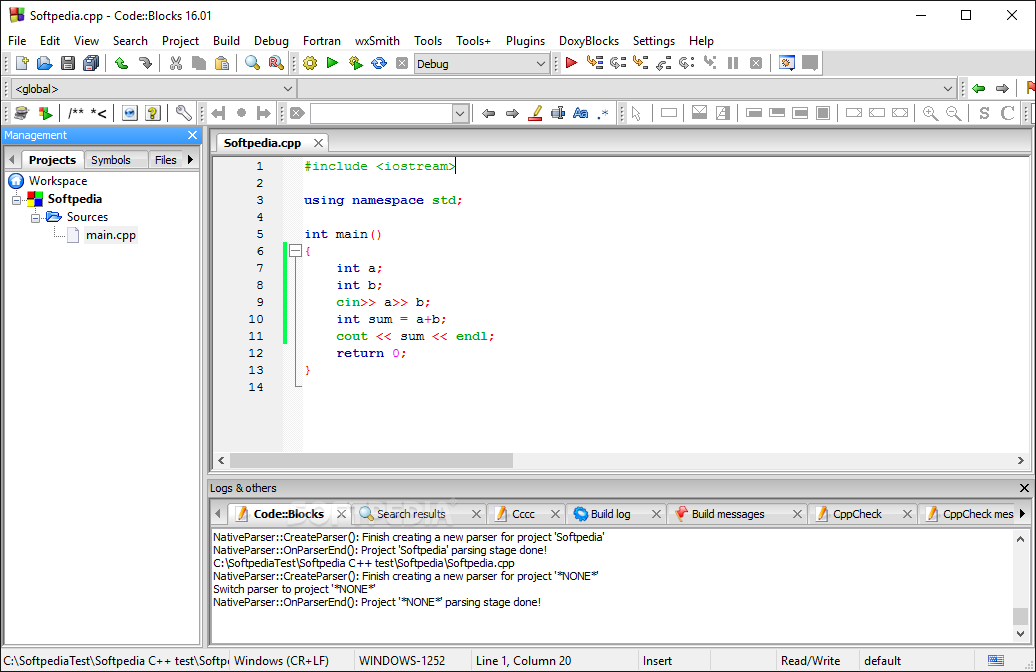
Exit In Dev C++
- C Programming Resources
- Selected Reading
Description
The C library function void exit(int status) terminates the calling process immediately. Any open file descriptors belonging to the process are closed and any children of the process are inherited by process 1, init, and the process parent is sent a SIGCHLD signal.
Exit Function In Dev C++
Declaration
Following is the declaration for exit() function.
Parameters
status − This is the status value returned to the parent process.
Customize in-game FPS for an incredibly seamless gaming performance. Cooking dash 3 pc download.
Return Value
This function does not return any value.
Example
The following example shows the usage of exit() function.
Let us compile and run the above program that will produce the following result −
In C++, you can exit a program in these ways:
- Call the exit function.
- Call the abort function.
- Execute a return statement from
main
.

exit function
The exit function, declared in <stdlib.h>, terminates a C++ program. The value supplied as an argument to exit
is returned to the operating system as the program's return code or exit code. By convention, a return code of zero means that the program completed successfully. You can use the constants EXIT_FAILURE and EXIT_SUCCESS, also defined in <stdlib.h>, to indicate success or failure of your program.
Issuing a return statement from the main
function is equivalent to calling the exit
function with the return value as its argument.
abort function
The abort function, also declared in the standard include file <stdlib.h>, terminates a C++ program. The difference between exit
and abort
is that exit
allows the C++ run-time termination processing to take place (global object destructors will be called), whereas abort
terminates the program immediately. The abort
function bypasses the normal destruction process for initialized global static objects. It also bypasses any special processing that was specified using the atexit function.
atexit function
How To Use Exit Function In C++
Use the atexit function to specify actions that execute prior to program termination. No global static objects initialized prior to the call to atexit are destroyed prior to execution of the exit-processing function.
return statement in main
Issuing a return statement from main
is functionally equivalent to calling the exit
function. Consider the following example:
The exit
and return statements in the preceding example are functionally identical. However, C++ requires that functions that have return types other than void return a value. The return statement allows you to return a value from main
.
Destruction of static objects
When you call exit
or execute a return statement from main
, static objects are destroyed in the reverse order of their initialization (after the call to atexit
if one exists). The following example shows how such initialization and cleanup works.
Example
In the following example, the static objects sd1
and sd2
are created and initialized before entry to main
. After this program terminates using the return statement, first sd2
is destroyed and then sd1
. The destructor for the ShowData
class closes the files associated with these static objects.
Another way to write this code is to declare the ShowData
objects with block scope, allowing them to be destroyed when they go out of scope: